mirror of
https://github.com/all-contributors/cli.git
synced 2025-01-10 05:56:29 +00:00
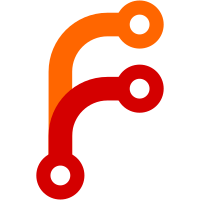
BREAKING CHANGE: Drop support for Node < v4. This uses native Promises available from Node v4. * fix: Bump inquirer to v3.0.1. Fixes #33 to improve Windows support. * refactor: Promisify everything as inquirer uses Promises from 1.0.0 onwards
37 lines
882 B
JavaScript
37 lines
882 B
JavaScript
'use strict';
|
|
|
|
var fs = require('fs');
|
|
var pify = require('pify');
|
|
var _ = require('lodash/fp');
|
|
|
|
function readConfig(configPath) {
|
|
try {
|
|
return JSON.parse(fs.readFileSync(configPath, 'utf-8'));
|
|
} catch (error) {
|
|
if (error.code === 'ENOENT') {
|
|
throw new Error('Configuration file not found: ' + configPath);
|
|
}
|
|
throw error;
|
|
}
|
|
}
|
|
|
|
function writeConfig(configPath, content) {
|
|
return pify(fs.writeFile)(configPath, JSON.stringify(content, null, 2) + '\n');
|
|
}
|
|
|
|
function writeContributors(configPath, contributors) {
|
|
var config;
|
|
try {
|
|
config = readConfig(configPath);
|
|
} catch (error) {
|
|
return Promise.reject(error);
|
|
}
|
|
var content = _.assign(config, {contributors: contributors});
|
|
return writeConfig(configPath, content);
|
|
}
|
|
|
|
module.exports = {
|
|
readConfig: readConfig,
|
|
writeConfig: writeConfig,
|
|
writeContributors: writeContributors
|
|
};
|