mirror of
https://github.com/all-contributors/cli.git
synced 2025-01-26 22:46:30 +00:00
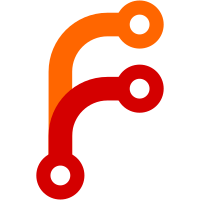
* feat: Add checking functionnality that compares contributors with GH data * fix(check): Use the info from config file, paginate GH data * doc(check): Document the new check command * fix(eslint): Add missing semicolon * tests: Add tests for utils.check * fix: Check for code and test only * refactor: use includes and correct awaits * refactor: more includes and template literals
45 lines
1,008 B
JavaScript
45 lines
1,008 B
JavaScript
'use strict';
|
|
|
|
var pify = require('pify');
|
|
var request = pify(require('request'));
|
|
|
|
function getNextLink(link) {
|
|
if (!link) {
|
|
return null;
|
|
}
|
|
|
|
var nextLink = link.split(',').find(s => s.includes('rel="next"'));
|
|
|
|
if (!nextLink) {
|
|
return null;
|
|
}
|
|
|
|
return nextLink.split(';')[0].slice(1, -1);
|
|
}
|
|
|
|
function getContributorsPage(url) {
|
|
return request.get({
|
|
url: url,
|
|
headers: {
|
|
'User-Agent': 'request'
|
|
}
|
|
})
|
|
.then(res => {
|
|
var body = JSON.parse(res.body);
|
|
var contributorsIds = body.map(contributor => contributor.login);
|
|
|
|
var nextLink = getNextLink(res.headers.link);
|
|
if (nextLink) {
|
|
return getContributorsPage(nextLink).then(nextContributors => {
|
|
return contributorsIds.concat(nextContributors);
|
|
});
|
|
}
|
|
|
|
return contributorsIds;
|
|
});
|
|
}
|
|
|
|
module.exports = function getContributorsFromGithub(owner, name) {
|
|
var url = `https://api.github.com/repos/${owner}/${name}/contributors?per_page=100`;
|
|
return getContributorsPage(url);
|
|
};
|