mirror of
https://github.com/all-contributors/cli.git
synced 2025-01-10 14:06:34 +00:00
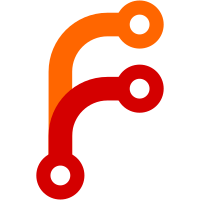
* Added exception handling when github returns 200s that have hit rate-limiting * Hardened the error check a bit
31 lines
709 B
JavaScript
31 lines
709 B
JavaScript
'use strict';
|
|
|
|
var pify = require('pify');
|
|
var request = pify(require('request'));
|
|
|
|
module.exports = function getUserInfo(username) {
|
|
return request.get({
|
|
url: 'https://api.github.com/users/' + username,
|
|
headers: {
|
|
'User-Agent': 'request'
|
|
}
|
|
})
|
|
.then(res => {
|
|
var body = JSON.parse(res.body);
|
|
var profile = body.blog || body.html_url;
|
|
|
|
// Github throwing specific errors as 200...
|
|
if (!profile && body.message) {
|
|
throw new Error(body.message);
|
|
}
|
|
|
|
profile = profile.startsWith('http') ? profile : 'http://' + profile;
|
|
|
|
return {
|
|
login: body.login,
|
|
name: body.name || username,
|
|
avatar_url: body.avatar_url,
|
|
profile
|
|
};
|
|
});
|
|
};
|