mirror of
https://github.com/renovatebot/renovate.git
synced 2025-01-12 15:06:27 +00:00
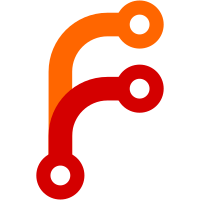
Adds support to run Renovate like “renovate owner/repo —dry-run”. Instead of creating branches, PRs or comments, an INFO level dry run message will be logged each time instead. Closes #1399
44 lines
1.5 KiB
JavaScript
44 lines
1.5 KiB
JavaScript
const { commitFilesToBranch } = require('../../../lib/workers/branch/commit');
|
|
const defaultConfig = require('../../../lib/config/defaults').getConfig();
|
|
|
|
describe('workers/branch/automerge', () => {
|
|
describe('commitFilesToBranch', () => {
|
|
let config;
|
|
beforeEach(() => {
|
|
config = {
|
|
...defaultConfig,
|
|
branchName: 'renovate/some-branch',
|
|
commitMessage: 'some commit message',
|
|
semanticCommits: false,
|
|
semanticCommitType: 'a',
|
|
semanticCommitScope: 'b',
|
|
updatedPackageFiles: [],
|
|
updatedLockFiles: [],
|
|
};
|
|
jest.resetAllMocks();
|
|
platform.commitFilesToBranch.mockReturnValueOnce('created');
|
|
});
|
|
it('handles empty files', async () => {
|
|
await commitFilesToBranch(config);
|
|
expect(platform.commitFilesToBranch.mock.calls.length).toBe(0);
|
|
});
|
|
it('commits files', async () => {
|
|
config.updatedPackageFiles.push({
|
|
name: 'package.json',
|
|
contents: 'some contents',
|
|
});
|
|
await commitFilesToBranch(config);
|
|
expect(platform.commitFilesToBranch.mock.calls.length).toBe(1);
|
|
expect(platform.commitFilesToBranch.mock.calls).toMatchSnapshot();
|
|
});
|
|
it('dry runs', async () => {
|
|
config.dryRun = true;
|
|
config.updatedPackageFiles.push({
|
|
name: 'package.json',
|
|
contents: 'some contents',
|
|
});
|
|
await commitFilesToBranch(config);
|
|
expect(platform.commitFilesToBranch.mock.calls.length).toBe(0);
|
|
});
|
|
});
|
|
});
|