mirror of
https://github.com/renovatebot/renovate.git
synced 2025-01-12 23:16:26 +00:00
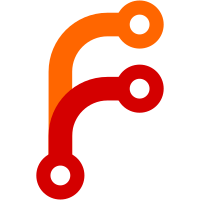
This is a major refactor of branch code to prepare for Yarn workspaces plus creating PRs for branches with failing lockfiles. Marked as "feature" to cause a minor version bump due to the moderate chance of accidentally breaking something.
52 lines
2.2 KiB
JavaScript
52 lines
2.2 KiB
JavaScript
const {
|
|
setUnpublishable,
|
|
} = require('../../../lib/workers/branch/status-checks');
|
|
const defaultConfig = require('../../../lib/config/defaults').getConfig();
|
|
const logger = require('../../_fixtures/logger');
|
|
|
|
describe('workers/branch/status-checks', () => {
|
|
describe('setUnpublishable', () => {
|
|
let config;
|
|
beforeEach(() => {
|
|
config = {
|
|
...defaultConfig,
|
|
api: { getBranchStatusCheck: jest.fn(), setBranchStatus: jest.fn() },
|
|
logger,
|
|
upgrades: [],
|
|
};
|
|
});
|
|
it('defaults to unpublishable', async () => {
|
|
await setUnpublishable(config);
|
|
expect(config.api.getBranchStatusCheck.mock.calls.length).toBe(1);
|
|
expect(config.api.setBranchStatus.mock.calls.length).toBe(0);
|
|
});
|
|
it('finds unpublishable true', async () => {
|
|
config.upgrades = [{ unpublishable: true }];
|
|
await setUnpublishable(config);
|
|
expect(config.api.getBranchStatusCheck.mock.calls.length).toBe(1);
|
|
expect(config.api.setBranchStatus.mock.calls.length).toBe(0);
|
|
});
|
|
it('removes status check', async () => {
|
|
config.upgrades = [{ unpublishable: true }];
|
|
config.api.getBranchStatusCheck.mockReturnValueOnce('pending');
|
|
await setUnpublishable(config);
|
|
expect(config.api.getBranchStatusCheck.mock.calls.length).toBe(1);
|
|
expect(config.api.setBranchStatus.mock.calls.length).toBe(1);
|
|
});
|
|
it('finds unpublishable false and sets status', async () => {
|
|
config.unpublishSafe = true;
|
|
config.upgrades = [{ unpublishable: true }, { unpublishable: false }];
|
|
await setUnpublishable(config);
|
|
expect(config.api.getBranchStatusCheck.mock.calls.length).toBe(1);
|
|
expect(config.api.setBranchStatus.mock.calls.length).toBe(1);
|
|
});
|
|
it('finds unpublishable false and skips status', async () => {
|
|
config.unpublishSafe = true;
|
|
config.upgrades = [{ unpublishable: true }, { unpublishable: false }];
|
|
config.api.getBranchStatusCheck.mockReturnValueOnce('pending');
|
|
await setUnpublishable(config);
|
|
expect(config.api.getBranchStatusCheck.mock.calls.length).toBe(1);
|
|
expect(config.api.setBranchStatus.mock.calls.length).toBe(0);
|
|
});
|
|
});
|
|
});
|