mirror of
https://github.com/renovatebot/renovate.git
synced 2025-01-15 09:06:25 +00:00
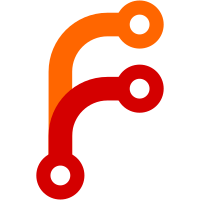
* refactor: strict null checks for util * chore: fix type * Update tsconfig.strict.json * Update lib/util/package-rules.ts * Update lib/util/package-rules.ts * chore: fix test and coverage * chore: fix package rules * refactor(manager): strict null checks * chore: revert config changes
84 lines
2.1 KiB
TypeScript
84 lines
2.1 KiB
TypeScript
import is from '@sindresorhus/is';
|
|
import { load } from 'js-yaml';
|
|
import { logger } from '../../../logger';
|
|
import { regEx } from '../../../util/regex';
|
|
import { HelmDatasource } from '../../datasource/helm';
|
|
import type { ExtractConfig, PackageDependency, PackageFile } from '../types';
|
|
import type { HelmsmanDocument } from './types';
|
|
|
|
const chartRegex = regEx('^(?<registryRef>[^/]*)/(?<packageName>[^/]*)$');
|
|
|
|
function createDep(
|
|
key: string,
|
|
doc: HelmsmanDocument
|
|
): PackageDependency | null {
|
|
const dep: PackageDependency = {
|
|
depName: key,
|
|
datasource: HelmDatasource.id,
|
|
};
|
|
const anApp = doc.apps[key];
|
|
if (!anApp) {
|
|
return null;
|
|
}
|
|
|
|
if (!anApp.version) {
|
|
dep.skipReason = 'no-version';
|
|
return dep;
|
|
}
|
|
dep.currentValue = anApp.version;
|
|
|
|
const regexResult = anApp.chart ? chartRegex.exec(anApp.chart) : null;
|
|
if (!regexResult?.groups) {
|
|
dep.skipReason = 'invalid-url';
|
|
return dep;
|
|
}
|
|
|
|
if (!is.nonEmptyString(regexResult.groups.packageName)) {
|
|
dep.skipReason = 'invalid-name';
|
|
return dep;
|
|
}
|
|
dep.packageName = regexResult.groups.packageName;
|
|
|
|
const registryUrl = doc.helmRepos[regexResult.groups.registryRef];
|
|
if (!is.nonEmptyString(registryUrl)) {
|
|
dep.skipReason = 'no-repository';
|
|
return dep;
|
|
}
|
|
dep.registryUrls = [registryUrl];
|
|
|
|
return dep;
|
|
}
|
|
|
|
export function extractPackageFile(
|
|
content: string,
|
|
fileName: string,
|
|
config: ExtractConfig
|
|
): PackageFile | null {
|
|
try {
|
|
// TODO: fix me (#9610)
|
|
const doc = load(content, {
|
|
json: true,
|
|
}) as HelmsmanDocument;
|
|
if (!(doc?.helmRepos && doc.apps)) {
|
|
logger.debug({ fileName }, 'Missing helmRepos and/or apps keys');
|
|
return null;
|
|
}
|
|
|
|
const deps = Object.keys(doc.apps)
|
|
.map((key) => createDep(key, doc))
|
|
.filter(is.truthy); // filter null values
|
|
|
|
if (deps.length === 0) {
|
|
return null;
|
|
}
|
|
|
|
return { deps };
|
|
} catch (err) /* istanbul ignore next */ {
|
|
if (err.stack?.startsWith('YAMLException:')) {
|
|
logger.debug({ err }, 'YAML exception extracting');
|
|
} else {
|
|
logger.warn({ err }, 'Error extracting');
|
|
}
|
|
return null;
|
|
}
|
|
}
|