mirror of
https://github.com/renovatebot/renovate.git
synced 2025-01-15 09:06:25 +00:00
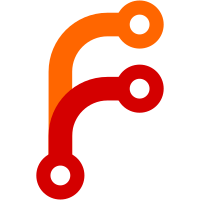
Renames `lookupName` to be `packageName`. BREAKING CHANGE: Use `packageName` instead of `lookupName` if interacting with Renovate datasources directly.
49 lines
1.5 KiB
TypeScript
49 lines
1.5 KiB
TypeScript
import { cache } from '../../../util/cache/package/decorator';
|
|
import { parseUrl } from '../../../util/url';
|
|
import * as rubyVersioning from '../../versioning/ruby';
|
|
import { Datasource } from '../datasource';
|
|
import type { GetReleasesConfig, ReleaseResult } from '../types';
|
|
import { InternalRubyGemsDatasource } from './get';
|
|
import { RubyGemsOrgDatasource } from './get-rubygems-org';
|
|
|
|
export class RubyGemsDatasource extends Datasource {
|
|
static readonly id = 'rubygems';
|
|
|
|
constructor() {
|
|
super(RubyGemsDatasource.id);
|
|
this.rubyGemsOrgDatasource = new RubyGemsOrgDatasource(
|
|
RubyGemsDatasource.id
|
|
);
|
|
this.internalRubyGemsDatasource = new InternalRubyGemsDatasource(
|
|
RubyGemsDatasource.id
|
|
);
|
|
}
|
|
|
|
override readonly defaultRegistryUrls = ['https://rubygems.org'];
|
|
|
|
override readonly defaultVersioning = rubyVersioning.id;
|
|
|
|
override readonly registryStrategy = 'hunt';
|
|
|
|
private readonly rubyGemsOrgDatasource: RubyGemsOrgDatasource;
|
|
|
|
private readonly internalRubyGemsDatasource: InternalRubyGemsDatasource;
|
|
|
|
@cache({
|
|
namespace: `datasource-${RubyGemsDatasource.id}`,
|
|
key: ({ registryUrl, packageName }: GetReleasesConfig) =>
|
|
`${registryUrl}/${packageName}`,
|
|
})
|
|
getReleases({
|
|
packageName,
|
|
registryUrl,
|
|
}: GetReleasesConfig): Promise<ReleaseResult | null> {
|
|
if (parseUrl(registryUrl)?.hostname === 'rubygems.org') {
|
|
return this.rubyGemsOrgDatasource.getReleases({ packageName });
|
|
}
|
|
return this.internalRubyGemsDatasource.getReleases({
|
|
packageName,
|
|
registryUrl,
|
|
});
|
|
}
|
|
}
|