mirror of
https://github.com/renovatebot/renovate.git
synced 2025-01-12 15:06:27 +00:00
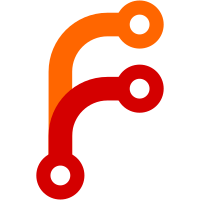
* Add jsonwebtoken * Add githubApp option * Add id and key configs * Add integer parsing to cli and env * Remove boolean app enablement * Drop got * Add API functions for GitHub Apps * debug not verbose in api * Add GitHub App Helper * Update redact function * Add GitHub app logic * Update logging * Fix lint * getInstallations tests * getInstallationToken tests * getInstallationRepositories tests * Refactor ghGot structure * Add github app tests * Add config tests * update lockfile
72 lines
1.8 KiB
JavaScript
72 lines
1.8 KiB
JavaScript
const commander = require('commander');
|
|
const configDefinitions = require('./definitions');
|
|
|
|
module.exports = {
|
|
getCliName,
|
|
getConfig,
|
|
};
|
|
|
|
function getCliName(option) {
|
|
if (option.cli === false) {
|
|
return '';
|
|
}
|
|
const nameWithHyphens = option.name.replace(/([A-Z])/g, '-$1');
|
|
return `--${nameWithHyphens.toLowerCase()}`;
|
|
}
|
|
|
|
function getConfig(argv) {
|
|
const options = configDefinitions.getOptions();
|
|
|
|
const config = {};
|
|
|
|
const coersions = {
|
|
boolean: val => val === 'true',
|
|
list: val => val.split(',').map(el => el.trim()),
|
|
string: val => val,
|
|
integer: val => parseInt(val, 10),
|
|
};
|
|
|
|
let program = new commander.Command().arguments('[repositories...]');
|
|
|
|
options.forEach(option => {
|
|
if (option.cli !== false) {
|
|
const param = `<${option.type}>`.replace('<boolean>', '[boolean]');
|
|
const optionString = `${getCliName(option)} ${param}`;
|
|
program = program.option(
|
|
optionString,
|
|
option.description,
|
|
coersions[option.type]
|
|
);
|
|
}
|
|
});
|
|
|
|
/* istanbul ignore next */
|
|
function helpConsole() {
|
|
/* eslint-disable no-console */
|
|
console.log(' Examples:');
|
|
console.log('');
|
|
console.log(' $ renovate --token abc123 singapore/lint-condo');
|
|
console.log(
|
|
' $ renovate --labels=renovate,dependency --ignore-unstable=false --log-level verbose singapore/lint-condo'
|
|
);
|
|
console.log(' $ renovate singapore/lint-condo singapore/package-test');
|
|
/* eslint-enable no-console */
|
|
}
|
|
|
|
program = program
|
|
.on('--help', helpConsole)
|
|
.action(repositories => {
|
|
config.repositories = repositories;
|
|
})
|
|
.parse(argv);
|
|
|
|
options.forEach(option => {
|
|
if (option.cli !== false) {
|
|
if (program[option.name] !== undefined) {
|
|
config[option.name] = program[option.name];
|
|
}
|
|
}
|
|
});
|
|
|
|
return config;
|
|
}
|