mirror of
https://github.com/renovatebot/renovate.git
synced 2025-01-13 15:36:25 +00:00
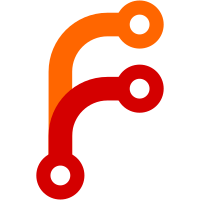
* refactor: introduce Fixtures util class * refactor: align yarn.spec * refactor: move fs mocks to __mocks__ folder * refactor: improve fs-extra mock * refactor: move callsite to dev deps * refactor: use fixtures class * refactor: use fixtures class * refactor: reduce external variables in tests * refactor: improve Fixtures * refactor: add type for realFs * refactor: remove obsolete snapshot * fix: by comments * refactor: provide ability to spy on mocked fs methods * refactor: fix build * refactor: fix by comments * refactor: add docs * refactor: fix unit test * refactor: return called times checks * refactor: fix by comments * refactor: adjust unit test * refactor: adjust unit test * refactor: fix unit test * refactor: fix by comments * refactor: fix by comments * refactor: update jsdoc * refactor: fix by comments Co-authored-by: Rhys Arkins <rhys@arkins.net> Co-authored-by: Jamie Magee <jamie.magee@gmail.com> Co-authored-by: Michael Kriese <michael.kriese@visualon.de>
62 lines
1.9 KiB
TypeScript
62 lines
1.9 KiB
TypeScript
import { Fixtures } from '../../../test/fixtures';
|
|
import { configFileNames } from '../../config/app-strings';
|
|
import { GlobalConfig } from '../../config/global';
|
|
import { EditorConfig } from './editor-config';
|
|
import { IndentationType } from './indentation-type';
|
|
|
|
jest.mock('fs');
|
|
const defaultConfigFile = configFileNames[0];
|
|
|
|
describe('util/json-writer/editor-config', () => {
|
|
beforeAll(() => {
|
|
GlobalConfig.set({
|
|
localDir: '',
|
|
});
|
|
});
|
|
|
|
beforeEach(() => {
|
|
Fixtures.reset();
|
|
});
|
|
|
|
it('should handle empty .editorconfig file', async () => {
|
|
expect.assertions(2);
|
|
Fixtures.mock({
|
|
'.editorconfig': '',
|
|
});
|
|
const format = await EditorConfig.getCodeFormat(defaultConfigFile);
|
|
|
|
expect(format.indentationSize).toBeUndefined();
|
|
expect(format.indentationType).toBeUndefined();
|
|
});
|
|
|
|
it('should handle global config from .editorconfig', async () => {
|
|
expect.assertions(2);
|
|
Fixtures.mock({
|
|
'.editorconfig': Fixtures.get('.global_editorconfig'),
|
|
});
|
|
const format = await EditorConfig.getCodeFormat(defaultConfigFile);
|
|
expect(format.indentationSize).toBe(6);
|
|
expect(format.indentationType).toBe(IndentationType.Space);
|
|
});
|
|
|
|
it('should not handle non json config from .editorconfig', async () => {
|
|
expect.assertions(2);
|
|
Fixtures.mock({
|
|
'.editorconfig': Fixtures.get('.non_json_editorconfig'),
|
|
});
|
|
const format = await EditorConfig.getCodeFormat(defaultConfigFile);
|
|
|
|
expect(format.indentationSize).toBeUndefined();
|
|
expect(format.indentationType).toBeUndefined();
|
|
});
|
|
|
|
it('should handle json config from .editorconfig', async () => {
|
|
expect.assertions(1);
|
|
Fixtures.mock({
|
|
'.editorconfig': Fixtures.get('.json_editorconfig'),
|
|
});
|
|
const format = await EditorConfig.getCodeFormat(defaultConfigFile);
|
|
|
|
expect(format.indentationType).toBe(IndentationType.Tab);
|
|
});
|
|
});
|