mirror of
https://github.com/renovatebot/renovate.git
synced 2025-01-15 09:06:25 +00:00
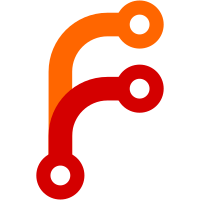
Adds gitFs support to Bitbucket Cloud. It is now mandatory to configure Bitbucket with username/password instead of token. Closes #2550, Closes #3024
80 lines
1.9 KiB
JavaScript
80 lines
1.9 KiB
JavaScript
const url = require('url');
|
|
const api = require('./bb-got-wrapper');
|
|
|
|
const repoInfoTransformer = repoInfoBody => ({
|
|
privateRepo: repoInfoBody.is_private,
|
|
isFork: !!repoInfoBody.parent,
|
|
repoFullName: repoInfoBody.full_name,
|
|
owner: repoInfoBody.owner.username,
|
|
mainbranch: repoInfoBody.mainbranch.name,
|
|
mergeMethod: 'merge',
|
|
});
|
|
|
|
const prStates = {
|
|
open: ['OPEN'],
|
|
notOpen: ['MERGED', 'DECLINED', 'SUPERSEDED'],
|
|
merged: ['MERGED'],
|
|
closed: ['DECLINED', 'SUPERSEDED'],
|
|
all: ['OPEN', 'MERGED', 'DECLINED', 'SUPERSEDED'],
|
|
};
|
|
|
|
const buildStates = {
|
|
success: 'SUCCESSFUL',
|
|
failed: 'FAILED',
|
|
pending: 'INPROGRESS',
|
|
};
|
|
|
|
const addMaxLength = (inputUrl, pagelen = 100) => {
|
|
const { search, ...parsedUrl } = url.parse(inputUrl, true);
|
|
const maxedUrl = url.format({
|
|
...parsedUrl,
|
|
query: { ...parsedUrl.query, pagelen },
|
|
});
|
|
return maxedUrl;
|
|
};
|
|
|
|
const accumulateValues = async (reqUrl, method = 'get', options, pagelen) => {
|
|
let accumulator = [];
|
|
let nextUrl = addMaxLength(reqUrl, pagelen);
|
|
const lowerCaseMethod = method.toLocaleLowerCase();
|
|
|
|
while (typeof nextUrl !== 'undefined') {
|
|
const { body } = await api[lowerCaseMethod](nextUrl, options);
|
|
accumulator = [...accumulator, ...body.values];
|
|
nextUrl = body.next;
|
|
}
|
|
|
|
return accumulator;
|
|
};
|
|
|
|
// istanbul ignore next
|
|
const isConflicted = files => {
|
|
for (const file of files) {
|
|
for (const chunk of file.chunks) {
|
|
for (const change of chunk.changes) {
|
|
if (change.content === '+=======') {
|
|
return true;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
return false;
|
|
};
|
|
|
|
const prInfo = pr => ({
|
|
number: pr.id,
|
|
body: pr.summary ? pr.summary.raw : undefined,
|
|
branchName: pr.source.branch.name,
|
|
title: pr.title,
|
|
state: prStates.closed.includes(pr.state) ? 'closed' : pr.state.toLowerCase(),
|
|
createdAt: pr.created_on,
|
|
});
|
|
|
|
module.exports = {
|
|
repoInfoTransformer,
|
|
prStates,
|
|
buildStates,
|
|
prInfo,
|
|
accumulateValues,
|
|
isConflicted,
|
|
};
|