mirror of
https://github.com/renovatebot/renovate.git
synced 2025-01-13 07:26:26 +00:00
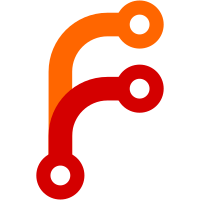
Renovate will now put all its data in `path.join(os.tmpdir(), '/renovate’);` and will instruct npm and yarn to do the same. To force Renovate to use a specific folder, set `process.env.TMPDIR` when running. The previous variable `RENOVATE_TMPDIR` is now deprecated and will be rewritten to TMPDIR. Closes #1794
58 lines
1.5 KiB
JavaScript
58 lines
1.5 KiB
JavaScript
const cacache = require('cacache/en');
|
|
const os = require('os');
|
|
const path = require('path');
|
|
const { DateTime } = require('luxon');
|
|
|
|
module.exports = {
|
|
init,
|
|
};
|
|
|
|
function getKey(namespace, key) {
|
|
return `${namespace}-${key}`;
|
|
}
|
|
|
|
const renovateCache = path.join(os.tmpdir(), '/renovate/renovate-cache-v1');
|
|
|
|
async function get(namespace, key) {
|
|
try {
|
|
const res = await cacache.get(renovateCache, getKey(namespace, key));
|
|
const cachedValue = JSON.parse(res.data.toString());
|
|
if (cachedValue) {
|
|
if (DateTime.local() < DateTime.fromISO(cachedValue.expiry)) {
|
|
logger.trace({ namespace, key }, 'Returning cached value');
|
|
return cachedValue.value;
|
|
}
|
|
// istanbul ignore next
|
|
await rm(namespace, key);
|
|
}
|
|
} catch (err) {
|
|
logger.trace({ namespace, key }, 'Cache miss');
|
|
}
|
|
return null;
|
|
}
|
|
|
|
async function set(namespace, key, value, ttlMinutes = 5) {
|
|
logger.trace({ namespace, key, ttlMinutes }, 'Saving cached value');
|
|
await cacache.put(
|
|
renovateCache,
|
|
getKey(namespace, key),
|
|
JSON.stringify({
|
|
value,
|
|
expiry: DateTime.local().plus({ minutes: ttlMinutes }),
|
|
})
|
|
);
|
|
}
|
|
|
|
// istanbul ignore next
|
|
async function rm(namespace, key) {
|
|
logger.trace({ namespace, key }, 'Removing cache entry');
|
|
await cacache.rm.entry(renovateCache, getKey(namespace, key));
|
|
}
|
|
|
|
async function rmAll() {
|
|
await cacache.rm.all(renovateCache);
|
|
}
|
|
|
|
function init() {
|
|
global.renovateCache = global.renovateCache || { get, set, rm, rmAll };
|
|
}
|