mirror of
https://github.com/renovatebot/renovate.git
synced 2025-01-14 16:46:25 +00:00
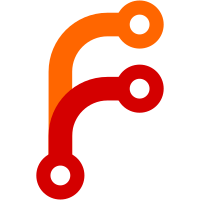
* feat(gitlabci-include): add support for 'include:local' * fix: lint * fix: apply suggestions from code review Co-authored-by: Michael Kriese <michael.kriese@visualon.de> * fix: lint * fix: includedDeps null check Co-authored-by: Michael Kriese <michael.kriese@visualon.de>
58 lines
1.8 KiB
TypeScript
58 lines
1.8 KiB
TypeScript
import fs from 'fs';
|
|
import { extractPackageFile } from './extract';
|
|
|
|
const yamlFile = fs.readFileSync(
|
|
'lib/manager/gitlabci-include/__fixtures__/gitlab-ci.1.yaml',
|
|
'utf8'
|
|
);
|
|
const yamlLocal = fs.readFileSync(
|
|
'lib/manager/gitlabci-include/__fixtures__/gitlab-ci.2.yaml',
|
|
'utf8'
|
|
);
|
|
|
|
const yamlLocalBlock = fs.readFileSync(
|
|
'lib/manager/gitlabci-include/__fixtures__/gitlab-ci.3.yaml',
|
|
'utf8'
|
|
);
|
|
|
|
describe('lib/manager/gitlabci-include/extract', () => {
|
|
describe('extractPackageFile()', () => {
|
|
it('returns null for empty', async () => {
|
|
expect(
|
|
await extractPackageFile('nothing here', '.gitlab-ci.yml', {})
|
|
).toBeNull();
|
|
});
|
|
it('extracts multiple include blocks', async () => {
|
|
const res = await extractPackageFile(yamlFile, '.gitlab-ci.yml', {});
|
|
expect(res.deps).toMatchSnapshot();
|
|
expect(res.deps).toHaveLength(3);
|
|
});
|
|
it('extracts local include block', async () => {
|
|
const res = await extractPackageFile(yamlLocal, '.gitlab-ci.yml', {});
|
|
expect(res.deps).toMatchSnapshot();
|
|
expect(res.deps).toHaveLength(1);
|
|
});
|
|
it('extracts multiple local include blocks', async () => {
|
|
const res = await extractPackageFile(
|
|
yamlLocalBlock,
|
|
'.gitlab-ci.yml',
|
|
{}
|
|
);
|
|
expect(res.deps).toMatchSnapshot();
|
|
expect(res.deps).toHaveLength(2);
|
|
});
|
|
it('normalizes configured endpoints', async () => {
|
|
const endpoints = [
|
|
'http://gitlab.test/api/v4',
|
|
'http://gitlab.test/api/v4/',
|
|
];
|
|
|
|
for (const endpoint of endpoints) {
|
|
const res = await extractPackageFile(yamlFile, '.gitlab-ci.yml', {
|
|
endpoint,
|
|
});
|
|
expect(res.deps[0].registryUrls[0]).toEqual('http://gitlab.test');
|
|
}
|
|
});
|
|
});
|
|
});
|